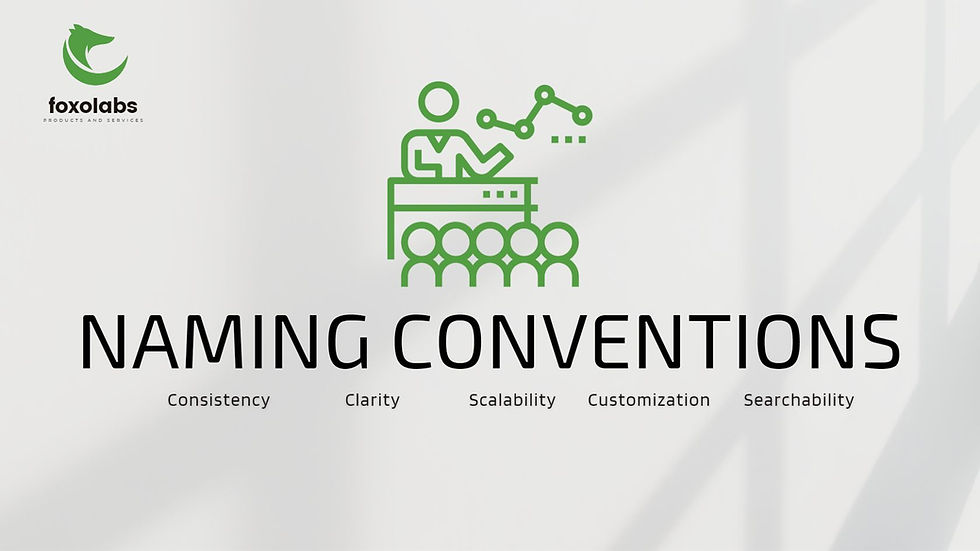
Why bother naming conventions in your product?
Consistency: Naming conventions ensure that all features, functions, and elements in the SaaS product have consistent and meaningful names. This makes it easier for users to navigate the product and understand its capabilities.
Clarity: A clear and consistent naming convention makes it easier for users to understand the function and purpose of each feature. This helps to reduce confusion and improve the user experience.
Scalability: Naming conventions allow SaaS products to scale and evolve over time. By using a consistent naming convention, new features and functions can be added to the product without disrupting the existing user experience.
Customization: Naming conventions allow users to customize their experience by creating their own names for features, functions, and elements within the SaaS product. This enhances the user experience and makes the product more personal.
Searchability: Naming conventions can improve the searchability of a SaaS product. By using consistent names and keywords, users can more easily find the features and functions they need. This can save time and improve the overall user experience.
Naming Conventions for Node JS
Naming conventions are important in Node.js projects to ensure that the code is easily understandable and maintainable. Here are some common naming conventions that are used in Node.js projects:
Packages: Packages should be named using lowercase letters and hyphens. For example, a package for a web framework could be named express.
Modules: Module names should be named using camelCase. For example, if you have a module for handling user authentication, it could be named userAuth.
Classes: Class names should be named using UpperCamelCase. For example, if you have a class for representing a user, it could be named User.
Functions: Function names should also be named using camelCase. For example, if you have a function for calculating the sum of two numbers, it could be named calculateSum.
Variables: Variable names should also be named using camelCase. For example, if you have a variable for storing a user's email address, it could be named userEmail.
Constants: Constants in Node.js should be named using all caps with underscores. For example, if you have a constant for the maximum number of login attempts, it could be named MAX_LOGIN_ATTEMPTS.
File names: File names for Node.js modules should be named using camelCase. For example, if you have a module for handling user authentication, the file name could be userAuth.js.
Events: When defining event handlers for a component, they should be named using camelCase and prefixed with "handle". For example, if you have an event handler for a button click, it could be named handleButtonClick.
Naming Conventions for React JS
Naming conventions are important in React to ensure that the code is easily understandable and maintainable. Here are some common naming conventions that are used in React:
Components: React components should be named using UpperCamelCase. For example, a component that displays a user profile could be named UserProfile.
Props: When defining props for a component, they should be named using camelCase. For example, if you have a prop for a user's name, it could be named userName.
State: State variables in a component should also be named using camelCase. For example, if you have a state variable that tracks the user's age, it could be named userAge.
Events: When defining event handlers for a component, they should be named using camelCase and prefixed with "handle". For example, if you have an event handler for a button click, it could be named handleButtonClick.
CSS classes: When defining CSS classes for a component, use lowercase with hyphens. For example, a class for a button could be named "button" or "primary-button".
It's important to be consistent in your naming conventions throughout your project. You can also use tools such as ESLint or Prettier to enforce naming conventions in your codebase. Additionally, you can create a style guide or document outlining your naming conventions for your team to follow.
Comments